Dependency Management Basics
Gradle has built-in support for dependency management.
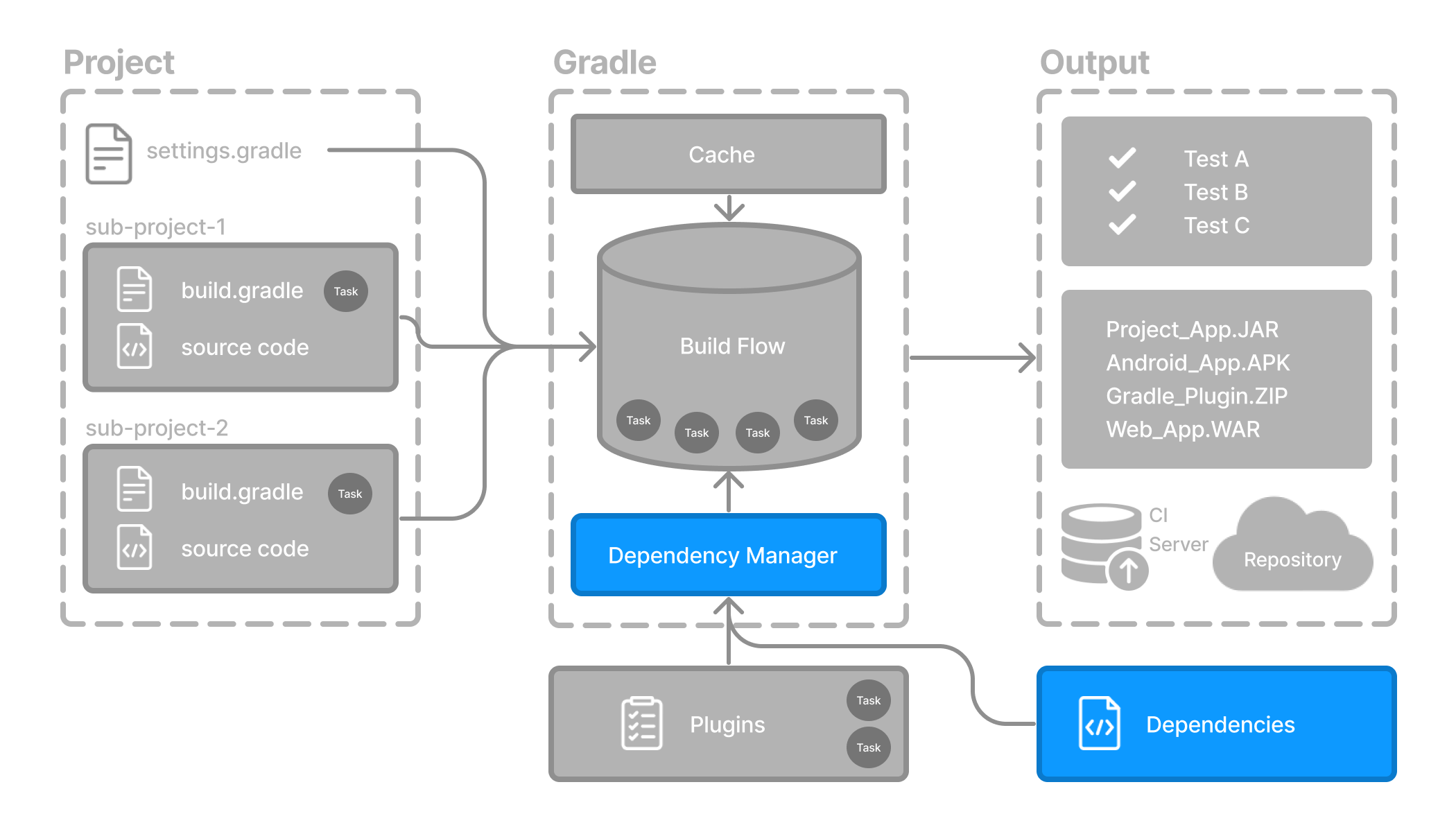
Dependency management is an automated technique for declaring and resolving external resources required by a project.
Gradle build scripts define the process to build projects that may require external dependencies. Dependencies refer to JARs, plugins, libraries, or source code that support building your project.
Version Catalog
Version catalogs provide a way to centralize your dependency declarations in a libs.versions.toml
file.
The catalog makes sharing dependencies and version configurations between subprojects simple. It also allows teams to enforce versions of libraries and plugins in large projects.
The version catalog typically contains four sections:
-
[versions] to declare the version numbers that plugins and libraries will reference.
-
[libraries] to define the libraries used in the build files.
-
[bundles] to define a set of dependencies.
-
[plugins] to define plugins.
[versions]
androidGradlePlugin = "7.4.1"
mockito = "2.16.0"
[libraries]
googleMaterial = { group = "com.google.android.material", name = "material", version = "1.1.0-alpha05" }
mockitoCore = { module = "org.mockito:mockito-core", version.ref = "mockito" }
[plugins]
androidApplication = { id = "com.android.application", version.ref = "androidGradlePlugin" }
The file is located in the gradle
directory so that it can be used by Gradle and IDEs automatically.
The version catalog should be checked into source control: gradle/libs.versions.toml
.
Declaring Your Dependencies
To add a dependency to your project, specify a dependency in the dependencies block of your build.gradle(.kts)
file.
The following build.gradle.kts
file adds a plugin and two dependencies to the project using the version catalog above:
plugins {
alias(libs.plugins.androidApplication) (1)
}
dependencies {
// Dependency on a remote binary to compile and run the code
implementation(libs.googleMaterial) (2)
// Dependency on a remote binary to compile and run the test code
testImplementation(libs.mockitoCore) (3)
}
1 | Applies the Android Gradle plugin to this project, which adds several features that are specific to building Android apps. |
2 | Adds the Material dependency to the project. Material Design provides components for creating a user interface in an Android App. This library will be used to compile and run the Kotlin source code in this project. |
3 | Adds the Mockito dependency to the project. Mockito is a mocking framework for testing Java code. This library will be used to compile and run the test source code in this project. |
Dependencies in Gradle are grouped by configurations.
-
The
material
library is added to theimplementation
configuration, which is used for compiling and running production code. -
The
mockito-core
library is added to thetestImplementation
configuration, which is used for compiling and running test code.
There are many more configurations available. |
Viewing Project Dependencies
You can view your dependency tree in the terminal using the ./gradlew :app:dependencies
command:
$ ./gradlew :app:dependencies
> Task :app:dependencies
------------------------------------------------------------
Project ':app'
------------------------------------------------------------
implementation - Implementation only dependencies for source set 'main'. (n)
\--- com.google.android.material:material:1.1.0-alpha05 (n)
testImplementation - Implementation only dependencies for source set 'test'. (n)
\--- org.mockito:mockito-core:2.16.0 (n)
...
Consult the Dependency Management chapter to learn more.
Next Step: Learn about Tasks >>